#TipTuesday Give a nudge to users who are still using the custom profile avatar.
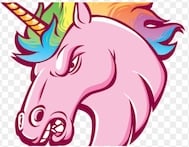

Here's an idea to add a personal touch to your community. I've created a custom HTML Widget to encourage users to complete their profiles with a snazzy avatar. Having custom photos/avatars help to bring out the personality of your community and encourage engagement. It's why give badges to users who upload their own photos.
This is a DIY widget that you will have to DIY (or you can DI with some help from someone from your team). It requires inserting a Custom HTML Widget in a Homepage Layout in the Appearance tab.
The idea is to detect if the current logged in user has a default avatar, and if so, show this message. If they have already uploaded a personal avatar or photo, don't show this message. It can look something like this, only cooler:
Here’s the simple recipe:
- Create a Custom HTML Widget: Decide where you want this friendly reminder to appear.
- Set Visibility for Admins: Initially, keep it under wraps from general users by setting the widget’s visibility to Administrators only.
- Craft Your Message: In the HTML panel, insert an HTML element where your message will live.
<!-- Custom HTML for Avatar Upload Prompt --> <div class="myMissingInfo hide" id="MyMissingInfo"> <h2 class="messagecontent title">Is this how you want to be seen?</h2> <div class="imageWrapper"><a href="/profile/picture" title="Click to update photo."><img src="" class="profilePhoto" id="profilePhoto"></a></div> <div class="messagecontent message"> I see you are using a default avatar. Don't be shy, show your true colors and help us know you better. </div> <div class="profileLinkWrapper"><a href="/profile/picture">Update Your Photo.</a></div> </div>
- Add Some JavaScript Magic: Use JavaScript to check if the profile is incomplete and then display the prompt.
// JavaScript to Check Profile Completion and Show Avatar Upload Prompt class UserProfile { constructor(e) { this.vanilla = e; this.shadowRoot = customHtmlRoot.shadowRoot; this.displayElementID = "MyMissingInfo"; this.displayElement = this.shadowRoot.getElementById(this.displayElementID) ?? false; this.currentUserId = this.vanilla.getCurrentUser().userID ?? 0; this.verifyUserProfile(); } verifyUserProfile = () => { // If the current user is a guest, abort. if (this.currentUserId === 0 ) { return; } // Do an API call to the current user's profile. this.vanilla.apiv2.get('/users/me').then((res) => { console.log(res.data); // Check if they current user has a default avatar... if (this.hasDefaultPhoto(res.data.photoUrl)) { // If so, display the avatar in the message, and make the message visible. this.displayCurrentPhoto(res.data.photoUrl); } }).catch((err) => {console.log('Looking for the UserProfile update message? It failed to load. Error message: ', err)}); } // Test for default photo or avatar. hasDefaultPhoto = (photo) => { if (photo.endsWith("defaulticon.png") || photo.includes("/uploads/defaultavatar/")) { return true; } return false; } // Display the current default avatar they are using in the message and set the message to show. displayCurrentPhoto = (photo) => { const imageTag = this.shadowRoot.getElementById('profilePhoto'); imageTag.src = photo; this.displayElement.classList.replace('hide', 'show'); } } onVanillaReady((e) => { new UserProfile(e); });
- Style with CSS: Make your prompt stand out with some CSS flair.
/* CSS for Avatar Upload Prompt */ .myMissingInfo { min-height: 200px; width: 100%; box-shadow: 1px 1px 3px 3px #eee; border-radius: 6px; padding: 15px 0; flex-direction: column; } .myMissingInfo h2.messagecontent.title { font-size: 20px; text-align: center; margin-bottom: 18px; } .myMissingInfo.hide { display: none; } .myMissingInfo.show { display: flex; } .messagecontent { display: flex; flex: 1; margin: 5px 15px; } .myMissingInfo .imageWrapper { position: relative; overflow: hidden; border-color: rgba(170, 173, 177, 0.3); border-width: 1px; border-style: solid; border-radius: 50%; margin: auto; max-height: 60px; max-width: 60px; } .imageWrapper img { max-height: 60px; max-width: 60px; } .messagecontent.warning { background-color: #fff8ce; padding: 6px 7px; } .profileLinkWrapper { width: 100%; margin: 30px auto; text-align: center; } .profileLinkWrapper a { padding: 5px 10px; border-width: 1px; border-style: solid; border-radius: 6px; text-decoration: none; margin: auto; } .profileLinkWrapper a { background-color: #037dbc; border-color: #037dbc; color: #ffffff; font-weight: 600; }
Remember, if coding isn’t your jam, there’s no harm in reaching out to a tech-savvy colleague to lend a hand. And don’t stress—keep it visible to just the admins while you fine-tune your creation. Once you’re ready to go live, update the conditions to share it with all your users.
If you have questions or comments, let me know below.
Happy coding, and here’s to a more connected community! 🚀
Comments
-
Agree, this is a great tip! Thanks so much for sharing @paddykelly
Tested it out in staging and it works a treat with a little editing to the colour code to match our site.
As an added benefit, it can also help with our gamification since members can earn the photogenic badge if they add a profile pic 📸
1 -
@Rav Singh Did you have to change anything else in the code besides the color? I tried adding the code in staging as is but it didn't work.
0 -
Hey @amorales
No, the only thing I changed was some of the messaging text and a couple of the colour hex codes to match our branding. Seemed to work ok in my case.
Note: We use a custom layout/layout editor so I created it using an HTML Widget in our custom layout as opposed to the Custom HTML pocket option in case that's what you're using?
0 -
Thank you everyone for your support. It does look like this is working for all of you but for some reason it is not working on mine. I'm using a custom HTML widget but I wonder if having the Vanillicon avatars as our default avatars enabled is a problem?
0 -
Well this widget seems to have been a bit of a success.
Judging by Photogenic badge log, we've moved from 2 or 3 average new Avatar users a day to having 100 in past few days.
Even seeing users that have been with us up to 20 years finally picking an Avatar!(Though I did change the code so it would be Pocket-ready so they're seeing it in every sidebar)
2 -
Hi @paddykelly
Hoping you might have some coding magic for this..
Is it possible to create a similar widget that targets members who haven't completed a specific custom profile field?
I'm hoping to replicate what you've created here with a widget that prompts members who haven't completed our 'My Software' custom profile field to do so. This will aid an automation rule that I'm planning to put in place for custom profile field ➡️ follow category.
0 -
Thank you @paddykelly for sharing this. And thank you, @amorales for the info about turning off the Vanillicon plugin. That was keeping mine from working as well.
1
Categories
- All Categories
- 23 Getting Started
- 438 Community Hub
- 354 Talk Community
- 63 Community Announcements & News
- What do you want to to do?
- Create an Account
- I'm new here
- Talk Higher Logic Vanilla
- Talk Community
- Check out the latest release
- Submit a product idea
- Get help
- View Events